Arrays, Why me?
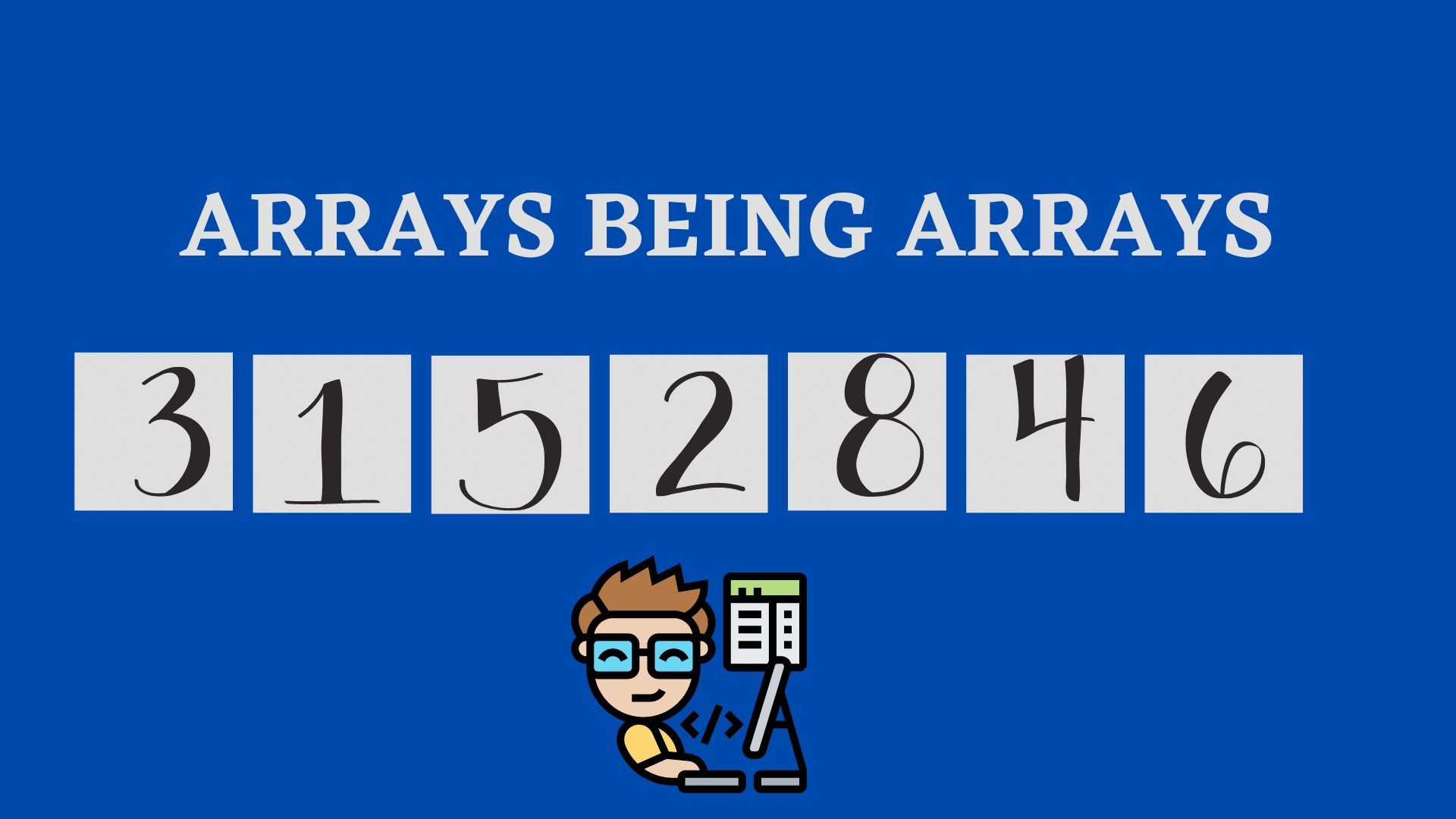
The blog is written for users who already have a basic understanding of arrays and need to solve questions in order to gain confidence in the subject.
What are Arrays?
WHY ARE ARRAYS
An array is a set of variables of the same data type stored in a list. This can be further explained with the following example:
Suppose a dummy has to store their marks in 7 tests in their code. They can either declare seven different variables named test_marks1,test_marks2,test_marks3... and so on OR simply declare an array of 7 variables containing marks of each of the test in whichever order they want.
This also clears why arrays are  important, They simply store large amount of values in a single variable. The given below code shows you how an array is declared for better understanding.
//Storing and printing values of Array
#include <iostream>
using namespace std;
void printarray(int arr[],int n) //store and print
{
cout<<"We have reached within print function."<<endl;
for(int i=0;i<15;i++)
{
arr[i]=i*2;
cout<<"num["<<i<<"] = "<<arr[i]<<endl;
}
cout<<"The values of array has been successfully printed!"<<endl;
}
int main() {
int num[15]; //declaration of array
cout<<"Command to print an array has been sent..."<<endl<<endl;
printarray(num,15);
cout<<"We have reached the end of the program.";
return 0;
}
In the rest of the blog, you will find two basic questions to get a better grasp at arrays and their working.
Ques 1.
Find the sum of all elements in an array.
Ans.
Let us break down the question and understand the logic. To find the sum of an array we would require a variable called sum. We will initialize and declare it to be '0' just so that a garbage value isn't automatically stored in it. Then, we will apply a for loop (as shown in the last blog) and skim through the array to traverse each element. The function of this particular for loop will be to take each element of the array and add it to our value of 'sum'. Hence, in the end when we give our sum as an output, we will have the sum of all elements in the array. Here is the code for better understanding.
//Storing, printing and also finding sum of elements in array
#include <iostream>
using namespace std;
void printarray(int arr[],int n)
{
cout<<"We have reached within print function."<<endl;
for(int i=0;i<15;i++)
{
arr[i]=1;
cout<<"num["<<i<<"] = "<<arr[i]<<endl;
}
cout<<"The values of array has been successfully printed!"<<endl;
}
void sumarr(int a[],int n)
{
int sum=0;
cout<<"We have entered the sum functions."<<endl;
for(int i=0;i<n;i++)
{
sum+=a[i];
}
cout<<"sum= "<<sum<<endl;
}
int main() {
int num[15];
cout<<"Command to print an array has been sent..."<<endl<<endl;
printarray(num,15);
sumarr(num,15);
cout<<"We have reached the end of the program.";
return 0;
}
Ques 2.
Find the minimum and maximum elements present in an array.
Ans.
To find the minimum value of an array, we will first have to declare an element called maximum. As the name suggests, it has to be a very large number (larger than any element stored in the array). We will then run a for loop to traverse the array. In this case, the function of this for loop will be to see if the element is lesser than maximum or not. If the value is lesser than the maximum, the value of the that particular element will be stored in maximum and the for loop will move forward to compare the next element with maximum. By the end of the loop, the minimum value element will be stored in the 'maximum' variable.
The exact same logic can be applied to find the maximum variable; where we take a variable called minimum and  compare it to each element in the array to see if it is larger than the stored value of minimum. In the end of the for loop, we obtain the maximum element present in array.
To further understand the concept, read the below code and try testing it with any array. Instead of running it on the laptop, use a pen and paper to first understand how the logic runs and then try it on your computer. Â
//Max and min values in array
#include <iostream>
using namespace std;
int getmax(int arr[],int n)
{
cout<<"We have entered the maximum value function."<<endl;
int max=-10000;
for(int i=0;i<n;i++)
{
if(arr[i]>max)
{
max=arr[i];
}
}
cout<<max;
return max;
}
int getmin(int A[],int n)
{
cout<<"We have entered the minimum value function."<<endl;
int min=10000;
for(int j=0;j<n;j++)
{
if(A[j]<min)
{
min=A[j];
}
}
cout<<min;
return min;
}
int main()
{
int n;
cout<<"PLEASE ENTER SIZE OF ARRAY: ";
cin>>n;
int a[n];
cout<<endl<<"Kindly enter elements of the array: ";
//taking input of array
for(int i=0;i<n;i++)
{
cin>>a[i];
}
cout<<endl<<"The max value in array is: "<<endl;
//get max function is called
getmax(a,n);
cout<<endl<<"The min value in array is: "<<endl;
//get min function is called
getmin(a,n);
return 0;
}
That is all for this blog. In the next few blogs, I will be sharing Leetcode solutions of several array related questions for better understanding of the subject.
Thank you for reading Dummies!