Before DSA
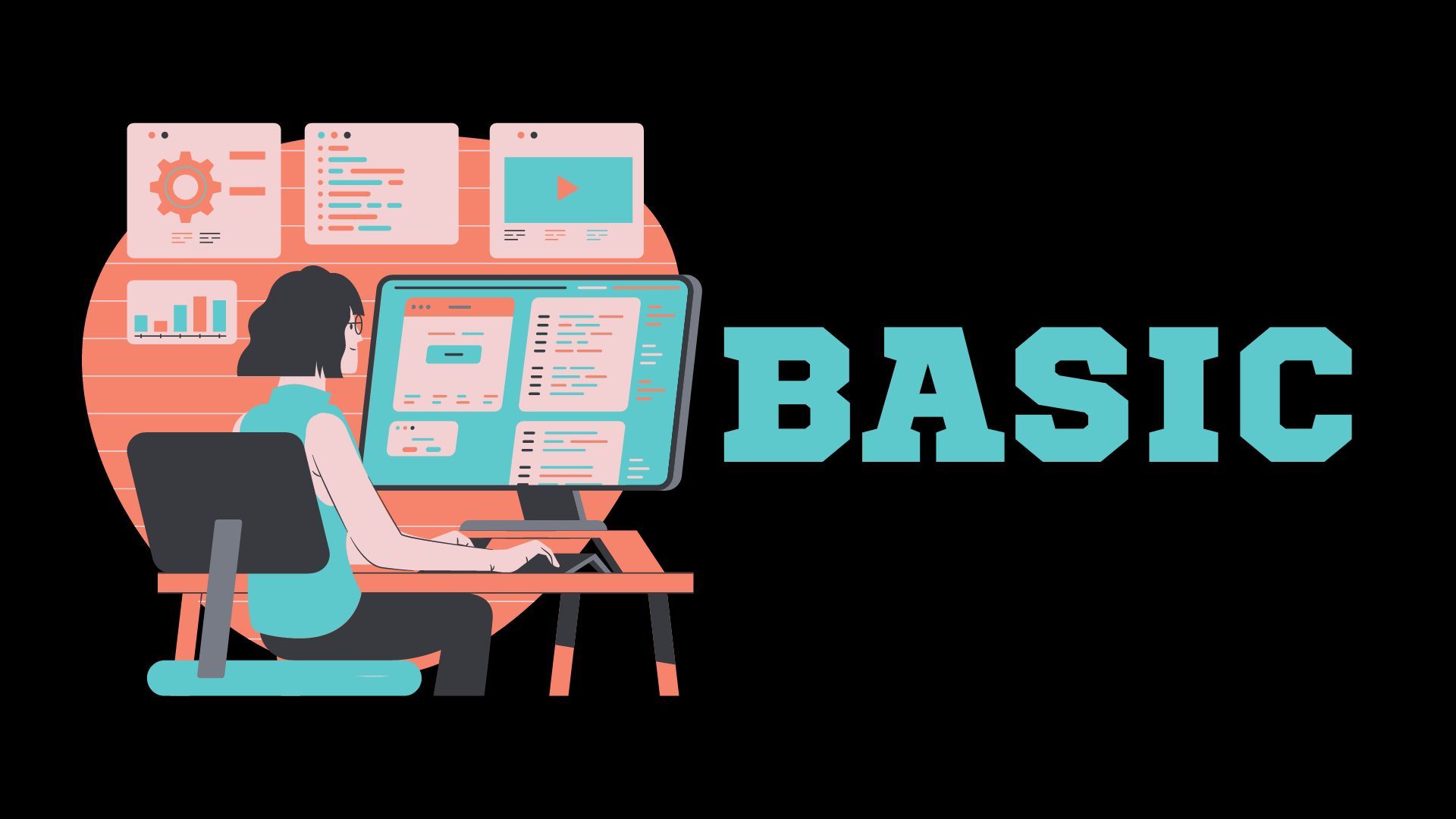
This blog is written for readers who already have a general understanding of C++ and want to revise some basic key concepts before starting with further topics.
Before moving to harder things, we must be clear with the basics of the language of our choice (C++ in this case). For this, we will overview some basic topics along with their questions. In this particular blog, you will find the topics:-
- If-Else Statements
- Loops (While and For)
- Operators
IF-ELSE STATEMENTS
Suppose we are given with a question, which follows two or more approaches based on specific conditions, we use if- else statements to determine outcomes based on given situations.
For example, a boy tosses a coin. If he gets heads, he goes to sleep and otherwise, he goes to code. In such a case, if a program is derived, where the boy inputs his outcome of toss and the code replies with what he will do in response, if else statements can be used.
Given below is an example code of if else for your understanding.
Question: Write a code to determine if the input character is an alphabet or a single digit number?
#include <iostream>
using namespace std;
int main()
{
char ch;
cout<<"Enter a single digit number or any single alphabet: ";
cin>>ch;
cout<<endl<<"Checking type of this character..."<<endl;
if ((ch>=65)&&(ch<=90))
{
cout<<"The character is an uppercase alphabet.";
}
else if((ch>=97)&&(ch<=122))
{
cout<<"character is a lowercase alphabet.";
}
else
{
cout<<"character is a single digit number.";
}
return 0;
}
LOOPS
A loop is made when a specific command has to be repeated a large of times in order to obtain the desired output. It is used to avoid making the code bulkier and to make it much easier for the coder to understand.
Given below are codes for application of while and for loops. Both the codes are basic level for understanding and syntax purposes only.
Question: Find the factorial of any number taken as input from user.
// FOR LOOP TO FIND FACTORIAL OF A NUMBER
#include <iostream>
using namespace std;
int main() {
int n,i;
int factorial=1;
cout<<"Enter a number to find it's factorial: ";
cin>>n;
for(i=1;i<=n;i++)
{
factorial=factorial*i;
}
cout<<endl<<factorial;
return 0;
}
In the above code, a variable i is made to increment from 1 to n (n is the value given by user). Since factorial of a number n!= n*(n-1)*(n-2)...*1 , hence values of i are multiplied (from 1 to n) and stored in the value of factorial to obtain the answer.
The same code can be implemented using While loop.
// WHILE LOOP TO FIND FACTORIAL OF A NUMBER
#include <iostream>
using namespace std;
int main() {
int n;
int i=1;
int factorial=1;
cout<<"Enter a number to find it's factorial: ";
cin>>n;
while(i<=n)
{
factorial=factorial*i;
i++;
}
cout<<endl<<factorial;
return 0;
}
OPERATORS
Operators are used in codes in order to to find the result of relationship between two conditions. Some important operators are:
- AND (&)
- OR(|)
- NOT(~)
- EX-OR(^)
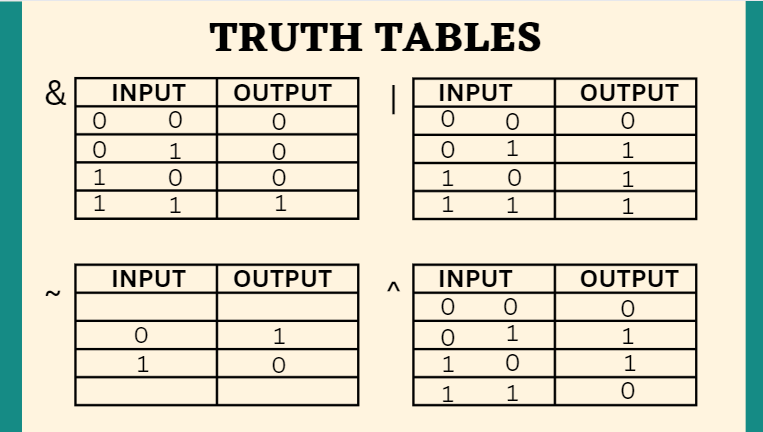
The code to find results of operations between two numbers is shown below.
//test operators
#include <iostream>
using namespace std;
int main()
{
int a=5;
int b=4;
cout<<"a= "<<a<<endl<<"b= "<<b<<endl;
cout<<"a AND b ="<<(a&b)<<endl;
cout<<"a OR b ="<<(a|b)<<endl;
cout<<"a NOT ="<< ~a<<endl;
cout<<"b NOT ="<<~b<<endl;
cout<<"a XOR b ="<<(a^b)<<endl;
return 0;
}
In this above code, both a and b are converted into their binary forms and then the operators are applied on each bit. This can be further depicted through the image given below.
Hence, some of the basic concepts that are important to cover before DSA have been discussed above. Other important concepts will be discussed according to the upcoming topics!
Happy Coding Dummies!